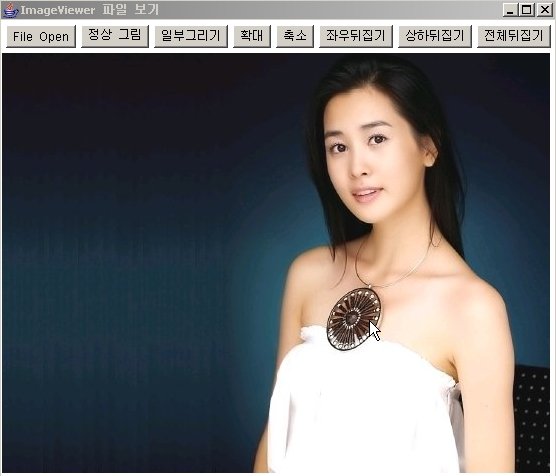
Image size : 556 Ⅹ 476
import java.awt.*;
import java.awt.image.*;
import java.awt.event.*;
import java.io.File;
import javax.swing.JOptionPane;
public class ImgViewer extends Frame implements ActionListener {
Image curImg;
Panel p;
Button b0, b1, b2, b3, b4, b5, b6, b7;
Dimension d;
int command;
static final int SHOW_NORM = 0;
static final int SHOW_PART = 1;
static final int SHOW_BIG = 2;
static final int SHOW_SMALL = 3;
static final int SHOW_HORIZONTAL = 4;
static final int SHOW_VERTICAL = 5;
static final int SHOW_ALL = 6;
ImgViewer(String s) {
super(s + " 파일 보기");
addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
dispose();
System.exit(0);
}
});
// curImg = Toolkit.getDefaultToolkit().getImage(s);
command = SHOW_NORM;
setLayout(new BorderLayout());
p = new Panel();
p.add(b0 = new Button("File Open")); // 파일 오픈 Button 추가
p.add(b1 = new Button("정상 그림"));
p.add(b2 = new Button("일부그리기"));
p.add(b3 = new Button("확대"));
p.add(b4 = new Button("축소"));
p.add(b5 = new Button("좌우뒤집기"));
p.add(b6 = new Button("상하뒤집기"));
p.add(b7 = new Button("전체뒤집기"));
add(p, BorderLayout.NORTH);
b0.addActionListener(this); // 해당 Button 에 ActionListener을 설정 한다.
b1.addActionListener(this);
b2.addActionListener(this);
b3.addActionListener(this);
b4.addActionListener(this);
b5.addActionListener(this);
b6.addActionListener(this);
b7.addActionListener(this);
setSize(500, 400);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
Button b = (Button) e.getSource();
if ( e.getSource() == b0 ) { // File Open Button 이 눌렸을 경우 처리...
openImage();
return;
} else if (b.getLabel().equals("정상 그림")) {
command = SHOW_NORM;
} else if (b.getLabel().equals("일부그리기")) {
command = SHOW_PART;
} else if (b.getLabel().equals("확대")) {
command = SHOW_BIG;
} else if (b.getLabel().equals("축소")) {
command = SHOW_SMALL;
} else if (b.getLabel().equals("좌우뒤집기")) {
command = SHOW_HORIZONTAL;
} else if (b.getLabel().equals("상하뒤집기")) {
command = SHOW_VERTICAL;
} else if (b.getLabel().equals("전체뒤집기")) {
command = SHOW_ALL;
}
reDraw(command);
}
// File Open Button 이 눌렸을 경우 호출 된다.
public void openImage() {
FileDialog fd = new FileDialog(this, "File Open");
fd.show();
String img = fd.getDirectory()+fd.getFile();
if ( isOpen(img) ) {
curImg = Toolkit.getDefaultToolkit().getImage(fd.getDirectory()+fd.getFile());
} else {
JOptionPane jp = new JOptionPane("Message Box", JOptionPane.ERROR_MESSAGE);
jp.showMessageDialog(this, "This is file not Image!! \nPlease !! Selected Image File ");
}
}
// 선택된 File이 이미지 File인지를 비교 true , false 를 Return
public boolean isOpen(String img) {
String[] extension = { "bmp", "jpg", "jpeg", "gif" };
for ( int i = 0; i < extension.length; i++) {
if ( img.toLowerCase().endsWith("."+extension[i])) {
return true;
}
}
return false;
}
/*
* int a=0;
*
* public boolean imageUpdate(Image img, int flags, int x, int y, int w, int
* h) { if((flags & ALLBITS) !=0) repaint();
*
* return (flags & (ALLBITS|ERROR)) == 0; }
*/
public void paint(Graphics g) {
if ( curImg == null ) return;
d = p.getSize();
int w = curImg.getWidth(this);
int h = curImg.getHeight(this);
switch (command) {
case SHOW_NORM:
g.drawImage(curImg, 5, d.height + 5, this);
break;
case SHOW_PART:
g.drawImage(curImg, 0, d.height, d.width / 2, d.height + d.width
/ 2, 0, 0, w / 2, h / 2, this);
break;
case SHOW_BIG:
g.drawImage(curImg, 0, d.height, 400, d.height + 400, 100, 100,
300, 300, this);
break;
case SHOW_SMALL:
g.drawImage(curImg, 0, d.height, w / 2, d.height + w / 2, 0, 0, w,
h, this);
break;
case SHOW_HORIZONTAL:
g.drawImage(curImg, 0, d.height, d.width, d.height + h, w, 0, 0, h,
this);
break;
case SHOW_VERTICAL:
g.drawImage(curImg, 0, d.height, d.width, d.height + h, w, h, 0, 0,
this);
break;
case SHOW_ALL:
g.drawImage(curImg, 0, d.height, d.width, d.height + h, 0, h, w, 0,
this);
}
}
public void reDraw(int command) {
this.command = command;
repaint();
}
public static void main(String args[]) {
ImgViewer iv = new ImgViewer("ImageViewer");
}
}
반응형
'Program' 카테고리의 다른 글
Balsamiq : 빠른 UI 디자인을 위한 도구 (0) | 2013.04.04 |
---|---|
이미지 크기에 맞게 새창띄우기 (0) | 2012.12.27 |
it관련 책 배포 사이트 (0) | 2012.12.14 |
Oracle - Date 날자 관련 함수 (0) | 2010.09.13 |
특정 프레임을 바꾸는 자바스크립트 (0) | 2010.09.09 |